Introduction
Deployment does not need to be hard or scary. You are a developer, and you can do it yourself. There are many ways of deploying a Laravel application, including multiple commercial offerings that make it much easier to deploy an application. In this article, I will show you how you can easily deploy your Laravel application to any server using a Dokku.
You will learn to create a database, create a Redis database, set up a Laravel app, link a domain to the app, secure the domain with Let's Encrypt, start a queue worker, and horizon.
First, why would you consider deploying an application yourself? You could do so to control your infrastructure, security, and performance, avoid vendor lock-in and ensure your code and data are portable across platforms, reduce costs, and save on costs.
About Dokku
Dokku is an open-source Platform as a Service (PAAS) alternative to Heroku. Docker powers it, and it is stable enough for production apps. Dokku assists in setting up applications, creating databases, linking domains, configuring web servers, securing domains with SSL, handling deployment, and scaling applications. These are standard actions, and Dokku automates them to save time.
There is no benefit in reinventing the wheel. With Linux knowledge, we could set up everything ourselves, but there is no benefit in reinventing the wheel. Once you set up the environment, all you will need to do is push code via git, and Dokku will zero-downtime deploy the application. Learn more from the Dokku website.
Prerequisites
To deploy a Laravel application with Dokku, you will need the following:
- The Laravel application that you want to deploy. The application must be version-controlled using the Git system.
- Server with a minimum of 1GB and either AMD64 (x86_64) or ARMV8 (arm64) architectures. You can get one from Digital Ocean using my referral link.
- A fresh installation of Ubuntu 20.04/22.04/24.04 or Debian 10+ x64 operating system. In this guide, we will use
Ubuntu
, my favourite. - You will need an SSH key on the computer you want to deploy from. There are many guides available on how to create an SSH key.
Step 1 of 9: Setup Dokku
The first step involves installing Dokku on the server. To do so, you will need a Username, IP Address and Password (optional). If you have not setup up SSH key on the server, you must copy the SSH keys to servers else you can skip this command
# e.g.ssh-copy-id [email protected]
ssh-copy-id username@IP
# e.g. ssh-copy-id -i ~/.ssh/dokku [email protected]
ssh-copy-id -i ~/.ssh/mykey username@IP
The authenticity of host '159.89.171.252 (159.89.171.252)' can't be established.
ED25519 key fingerprint is SHA256:Ndc...6agqwE8GH®.
This key is not known by any other names.
Are you sure you want to continue connecting (yes/no/[fingerprint])? yes
/usr/bin/ssh-copy-id: INFO: attempting to log in with the new key(s), to filter out any that are already installed /usr/bin/ssh-copy-id: INFO: 1 key(s) remain to be installed -- if you are prompted now it is to install the new keys
[email protected]'s password:
Number of key(s) added:
1
Now try logging into the machine, with:
"ssh '[email protected]'"
and check to make sure that only the keys) you wanted were added.
The ssh-copy-id command logs into the server host, copies keys to the server, and configures them to grant access by adding them to the authorized_keys file. The copying may ask for a password
or other authentication for the server.
When your server ssh key is set up, log in into the server:
# e.g. ssh [email protected]
ssh root@IP
# e.g ssh -i ~/.ssh/dokku [email protected]
ssh -i ~/.ssh/dokku [email protected]
Once logged in, start by making sure your server packages are up-to-date. Updating the packages is essential to ensure system security, stability, and access to the latest features and bug fixes.
sudo apt-get update
sudo apt-get upgrade
After that, install the latest stable version of Dokku. The version could be different.
wget -NP . https://dokku.com/install/v0.35.3/bootstrap.sh
sudo DOKKU_TAG=v0.35.3 bash bootstrap.sh
The installation may take 5-10 minutes, depending on your internet connection. You could use the time for a coffee break.
The last step in Dokku installation is to allow the listed keys to access the Dokku instance as the admin user via SSH. Without this, you cannot push your code to the Dokku in the server.
cat ~/.ssh/authorized_keys | dokku ssh-keys:add admin
Once installed, you should verify the installation:
shell dokku version
shell dokku version 0.35.5
Here is a video demonstrating the entire Dokku setup process on Ubuntu.
Step 2 of 9: Setup a Database
To setup your application database, install the respective Dokku plugin. You can use MariaDB, PostgreSQL or MySQL.
SSH into your server and install the database plugin of your choice. We will use PostgreSQL in this guide, but you can use MariaDB or MySQL.
sudo dokku plugin:install https://github.com/dokku/dokku-postgres.git postgres
Next, we create a postgres database for the application, let's call it laravel-postgres
.
dokku postgres:create laravel-postgres
# You can specify the image name and version
# as long as it is postgres compatible.
dokku postgres:create laravel-postgres \
--image "postgres" \
--image-version "16.4-alpine"
Within a few minutes, the postgres:create
will spin up a new postgres container
and create a database
with a user
with a secure password
. When the command is finished, you will see the database information, including DATABASE_URL,
local storage folder,
and other information.
Since the container data is temporary, the plugin will store database data in the /var/lib/dokku/services/postgres/laravel-postgres
directory on the server. That way, your database data will remain intact even if the container is detected. You can view the database information by running the info command.
dokku postgres:info laravel-postgres
=====> laravel-postgres postgres service information
Config dir: /var/lib/dokku/services/postgres/laravel-postgres/data
Config options:
Data dir: /var/lib/dokku/services/postgres/laravel-postgres/data
Dsn: postgres://postgres:c356...808e@dokku-postgres-laravel-postgres:5432/laravel_postgres
Exposed ports:
Id: 2cd276b13d06...ded2699014f454c0e
Internal ip: 172.17.0.2
Initial network:
Links: -
Post create network:
Post start network:
Service root: /var/lib/dokku/services/postgres/laravel-postgres
Status: running
Version: postgres:17.0
In the output, note the Dsn
field. This is the database connection string that we will inject into our Laravel application and connect to the database.
At this point, the database is ready for use. You can verify it by entering and interacting with the database inside the container using the psql
command.
dokku postgres:enter laravel-postgres
If you wish to access the database from outside the container, you can expose the port to the host.
# Choose a random port e.g. 2976
dokku postgres:expose laravel-postgres 2976
If you expose the port to the host, ensure you add a new
firewall rule
to allow access to the database from outside the container. e.g. a firewall rule that allows access from a specific IP address.
Here is a video demonstrating the process of setting up Postgres using Dokku.
Step 3 of 9: Setup a Redis
To improve the performance of the Laravel application and power horizon, we will use Redis. We start by installing the Redis plug-in for Dokku.
sudo dokku plugin:install https://github.com/dokku/dokku-redis.git redis
Next, we create a Redis instance for the application; let's call it laravel-redis
.
dokku redis:create laravel-redis
# You can specify the image name and version
dokku redis:create laravel-redis \
--image "redis" \
--image-version "7.4.0-bookworm"
Within a few minutes, the redis:create
will spin up a new redis container
and create a secure password
. When the command is finished, you will see the database information, including REDIS_URL,
data storage folder,
and other information.
Since the container data is temporary, the plugin will store database data in the /var/lib/dokku/services/redis/laravel-redis
directory. You can view the database information by running the info command.
dokku redis:info laravel-redis
=====> laravel-redis redis service information
Config dir: /var/lib/dokku/services/redis/laravel-redis/config
Config options:
Data dir: /var/lib/dokku/services/redis/laravel-redis/data
Dsn: redis://:b563e6ff6...bcb91139f1acebd8@dokku-redis-laravel-redis:6379
Exposed ports:
Id: eb720773d0.....709b6fd7
Internal ip: 172.17.0.4
Initial network:
Links: laravel-app
Post create network:
Post start network:
Service root: /var/lib/dokku/services/redis/laravel-redis
Status: running
Version: redis:7.2.5
In the output, note the Dsn
field. This is the database connection string that we will inject into our Laravel application and connect to the database.
At this point, the redis is ready for use. You can verify it by entering and interacting with the redis inside the redis container using the redis-cli
command.
dokku postgres:enter laravel-postgres
If you wish to access the redis database from outside the container, you can expose the port to the host.
# Choose a random port e.g. 6920
dokku redis:expose laravel-redis 6920
If you expose the port to the host, ensure you add a new
firewall rule
to allow access to the redis from outside the container. e.g. a firewall rule that allows access from a specific IP address.
Here is a video demonstrating Redis's entire setup process using Dokku.
Step 4 of 9: Create Laravel App
Creating a new Laravel application is as simple as running dokku apps:create
command. Let's call our app laravel-app
.
dokku apps:create laravel-app
-----> Creating laravel-app... -----> Creating new app virtual host file...
Next we need to link postgres database to the app. Linking ensure that 'DATABASE_URL' environment variable is available to the laravel app.
dokku postgres:link laravel-postgres laravel-app
shell -----> Setting config vars DATABASE_URL:
postgres://postgres:c35..808e@dokku-postgres-laravel-postgres:5432/laravel_postgres -----> Restarting app
laravel-app ! App image (dokku/laravel-app:latest) not found
Next we need to link redis database to the app. Linking ensure that 'REDIS_URL' environment variable is available to the laravel app.
dokku redis:link laravel-redis laravel-app
-----> Setting config vars REDIS_URL: redis://:b563e6...ebd8@dokku-redis-laravel-redis:6379 ----->
Restarting app laravel-app ! App image (dokku/laravel-app:latest) not found
Linking is simply a way of networking the database container to the app container in a network. This way, the app can access the database in a secure network.
Now that we hav setup DATABASE_URL
and REDIS_URL
environment variables, we can set the remaining environment variables..
dokku config:set --no-restart laravel-app \
APP_NAME="Laravel App on Dokku" \
APP_KEY=base64:PVGsYQYCoqtE5cRuTKX8TDCaZzK1bE0UrkNm3ezpkR0= \
DB_CONNECTION=pgsql \
APP_ENV=production \
APP_DEBUG=false \
APP_TIMEZONE=Africa/Nairobi \
APP_URL=https://demo.artisanelevated.com \
BCRYPT_ROUNDS=12 \
SESSION_DRIVER=redis \
BROADCAST_CONNECTION=redis \
QUEUE_CONNECTION=redis \
CACHE_STORE=redis \
PHP_OPCACHE_ENABLE=1
Generate the laravel application key locally using
php artisan key:generate --show
We are using config:set
because we will not have a chance to create a .env
file as you may be used to in Laravel. You can set as many environment variables as you want. Confirm that the environment variable has been set by displaying all the app environment variables.
shell dokku config:show laravel-app
APP_DEBUG: false APP_ENV: production APP_KEY: base64:PVGsYQYCoqtE5cRuTKX8TDCaZzK1bE0UrkNm3ezpkR0=
APP_NAME: Laravel App on Dokku APP_TIMEZONE: Africa/Nairobi APP_URL: https://demo.artisanelevated.com
BCRYPT_ROUNDS: 12 BROADCAST_CONNECTION: redis CACHE_STORE: redis DB_CONNECTION: pgsql DATABASE_URL:
postgres://postgres:c3..8e@dokku-postgres-laravel-postgres:5432/laravel_postgres PHP_OPCACHE_ENABLE: 1
QUEUE_CONNECTION: redis REDIS_URL: redis://:b563..cebd8@dokku-redis-laravel-redis:6379 SESSION_DRIVER: redis
At this point, the dokku laravel-app
us ready for your code
Step 5 of 9: Prepare the Laravel Application
To deploy a Laravel application with Dokku, we need to make a few changes.
5.1 Rename DB_URL
to DATABASE_URL
Start by opening /config/database.php
and renaming DB_URL
to DATABASE_URL
. This ensures that the Laravel can connect to the database.
// config/database.php
return [
'connections' => [
'mysql' => [
'url' => env('DATABASE_URL'),
# ...
],
'mariadb' => [
'url' => env('DATABASE_URL'),
# ...
],
'pgsql' => [
'url' => env('DATABASE_URL'),
# ...
],
],
];
5.2 Create a Dockerfile
Next, we need to create a Dockerfile
at the application's root. Dokku uses this file to build the application's image.
cd /your/lacal/path/to/laravel-app
touch Dockerfile
Dokku support other build methods that do not require a
Dockerfile
. However, I preferDockerfile
as it gives us more control over the build process.
We will use Serversideup PHP docker images. These images are built on top of the official PHP docker images. They are production-ready, high-performing, customizable, available in multiple flavours, and open source. However, you are free to use any image you want.
Here is a custom Dockerfile
that I usually use. It includes the additional step of installing NodeJS and building the application. You can remove the node part if you don't need it.
# Select the variant that you want from
# https://serversideup.net/open-source/docker-php/
FROM serversideup/php:8.3-unit
# Specify the NodeJS version
ARG NODE_VERSION=20
# Allow HTTP and HTTPS.
ENV SSL_MODE=mixed
# Switch to root so we can install NodeJS
# to the base image
USER root
# Install the intl extension for translations
RUN install-php-extensions exif
# Install NodeJS -> remove this if you do not need NodeJS
RUN apt-get update \
&& apt-get install -y bash \
&& curl -fsSL https://deb.nodesource.com/setup_$NODE_VERSION.x -o nodesource_setup.sh \
&& bash nodesource_setup.sh \
&& apt-get install -y nodejs \
&& apt-get install -y jpegoptim optipng pngquant gifsicle libavif-bin \
&& npm install -g svgo \
&& apt-get -y autoremove \
&& apt-get clean \
&& rm -rf /var/lib/apt/lists/* /tmp/* /var/tmp/* /usr/share/doc/*
# Switch back to non-privellage user www-data user
USER www-data
# Install production composer dependencies
COPY --chown=www-data:www-data composer.json composer.lock ./
RUN composer install --no-dev --prefer-dist --no-scripts --no-autoloader --no-progress --ignore-platform-reqs
# Install node dependencies -> remove this if you do not need NodeJS
COPY --chown=www-data:www-data package.json ./
RUN npm install --frozen-lockfile \
&& npm run build
# Copy the app files to the container
COPY --chown=www-data:www-data . .
# Autoload files
RUN composer dump-autoload --optimize
# Prepare the laravel app
RUN php /var/www/html/artisan storage:link
I recommend you consider a container that has a web server such as unitd, nginx + php-fpm, or apache + php-fpm. You can use any of them. I am using unitd because it is lightweight, high performing and reliable.
5.3 Create a Procfile
Next we need to create a Procfile
at the root of the application. This file is used by Dokku to start the application.
cd /your/lacal/path/to/laravel-app
touch Procfile
Then we need to specify the command to run the application in the Procfile
.
web: unitd --no-daemon
scheduler: php /var/www/html/artisan schedule:work
horizon: php /var/www/html/artisan horizon
reverb: php /var/www/html/artisan reverb:start
release: php /var/www/html/artisan migrate --force
Dokku app run on a container. Replace
/var/www/html/
with the path to your Laravel application.
Let me explain what each command does:
web
: A mandatory command to start the application. In this case weunitd --no-daemon
to start a dynamic application server for our Laravel application. If you are using other web servers, the command will be different. e.g.php-fpm
,horizon
: This is the command to start the horizon worker. If you are not using horizon, you can remove this command and replace it withphp /var/www/html/artisan queue:work --tries=3
.scheduler
: This is the command to start the scheduler worker.websocket
: This is the command to start the websocket worker for handling websocket connections. If you are not using revert, replace with the right command.release
: This is not a process. It is a command that is executed after the laravel-app's docker image is built, but before any containers are scheduled.
You can ignore horizon
, scheduler
and websocket
if you do not need them. Dokku will spin up a container for each of the services as we will see later.
5.4 Create a app.json
file (optional)
Finally in the app preparation step, we will create a app.json
file. This file is used by dokku to configure the application. app.json
is not mandatory, but it is useful for configuring dokku.
For example, to ensure that Dokku performs zero downtime deployments
, we need to specify the healthchecks
points for our processes. When Dokku health checks pass, the respective container will be deployed, and the old container will be shut down in 60s. If it fails, the application will not be deployed, and the old container will be kept running.
{
"scripts": {
"dokku": {
"predeploy": "php /var/www/html/artisan optimize:clear",
"postdeploy": "php /var/www/html/artisan optimize"
},
"postdeploy": ""
},
"healthchecks": {
"web": [
{
"name": "app-health",
"description": "Checking if the laravel app container is up and running.",
"type": "startup",
"port": 8080,
"uptime": 10,
"timeout": 20
}
],
"scheduler": [
{
"name": "scheduler-health",
"description": "Checking if the laravel scheduler container is up and running.",
"type": "startup",
"command": ["php", "/var/www/html/artisan", "schedule:test", "--name=inspire"],
"timeout": 10
}
],
"horizon": [
{
"name": "horizon-health",
"description": "Checking if the laravel horizon container is up and running.",
"type": "startup",
"command": ["php", "/var/www/html/artisan", "horizon:status"],
"timeout": 10
}
]
}
}
Make sure you commit the changes.
Step 6 of 9: Your First Deployment
Dokku allow you to push code straight from your repository to the server. To do so, you need to add a remote to your git repository.
shell git remote add dokku dokku@IP_ADDRESS:laravel-app git remote -v
https://github.com/njoguamos/laravel-dokku.git (fetch) origin https://github.com/njoguamos/laravel-dokku.git
(push) ```
</Tab>
</Tabs>
Next we deploy the main branch to the server.
<Tabs>
<Tab name="deploy-main" label="Deploy" checked>
```shell
git push dokku main
Enumerating objects: 85, done.
Counting objects: 100% (85/85), done.
Delta compression using up to 8 threads
Compressing objects: 100% (68/68), done.
Writing objects: 100% (85/85), 63.66 KiB | 5.30 MiB/s, done.
Total 85 (delta 8), reused 0 (delta 0), pack-reused 0 (from 0)
-----> Set main as deploy-branch
-----> Cleaning up...
-----> Building laravel-app from Dockerfile
remote: # Docker build output
-----> Releasing laravel-app...
-----> Checking for predeploy task
No predeploy task found, skipping
-----> Checking for release task
Executing release task from Procfile in ephemeral container: php artisan migrate --force && php artisan horizon:terminate
=====> Start of laravel-app release task (cde038047) output
=====> End of laravel-app release task (cde038047) output
-----> Checking for first deploy postdeploy task
No first deploy postdeploy task found, skipping
For more efficient zero downtime deployments, add healthchecks to your app.json. See https://dokku.com/docs/deployment/zero-downtime-deploys/ for examples
-----> Deploying laravel-app via the docker-local scheduler...
-----> Deploying web (count=1)
=====> Triggering early nginx proxy rebuild
-----> Ensuring network configuration is in sync for laravel-app
-----> Configuring laravel-app.ubuntu-s-1vcpu-1gb-35gb-intel-blr1-01...(using built-in template)
-----> Creating http nginx.conf
Reloading nginx
-----> Deploying release (count=0)
-----> Deploying scheduler (count=0)
-----> Running post-deploy
-----> Ensuring network configuration is in sync for laravel-app
-----> Configuring laravel-app.ubuntu-s-1vcpu-1gb-35gb-intel-blr1-01...(using built-in template)
-----> Creating http nginx.conf
Reloading nginx
-----> Renaming containers
Renaming container laravel-app.web.1.upcoming-30059 (7d7ff84276e9) to laravel-app.web.1
-----> Checking for postdeploy task
No postdeploy task found, skipping
=====> Application deployed:
http://laravel-app.ubuntu-s-1vcpu-1gb-35gb-intel-blr1-01
To 159.89.171.252:laravel-app
* [new branch] main -> main
If there was no error, the application was deployed successfully. But not accessible yet. Let's fix that.
Step 7 of 9: Add a domain to the app
We need to be able to access the application from the internet. To do so, we need to add a domain to the app. Navigate to your domain provider and point the domain to the IP address of the server using an A record
.
If you use Cloudflare like me, turn off the DNS-only
option. Else, you may not be able to add a free ssl certificate later.
In this case, I will point subdomain, demo.artisanelevated.com
to the IP address (159.89.171.252)
of the server.
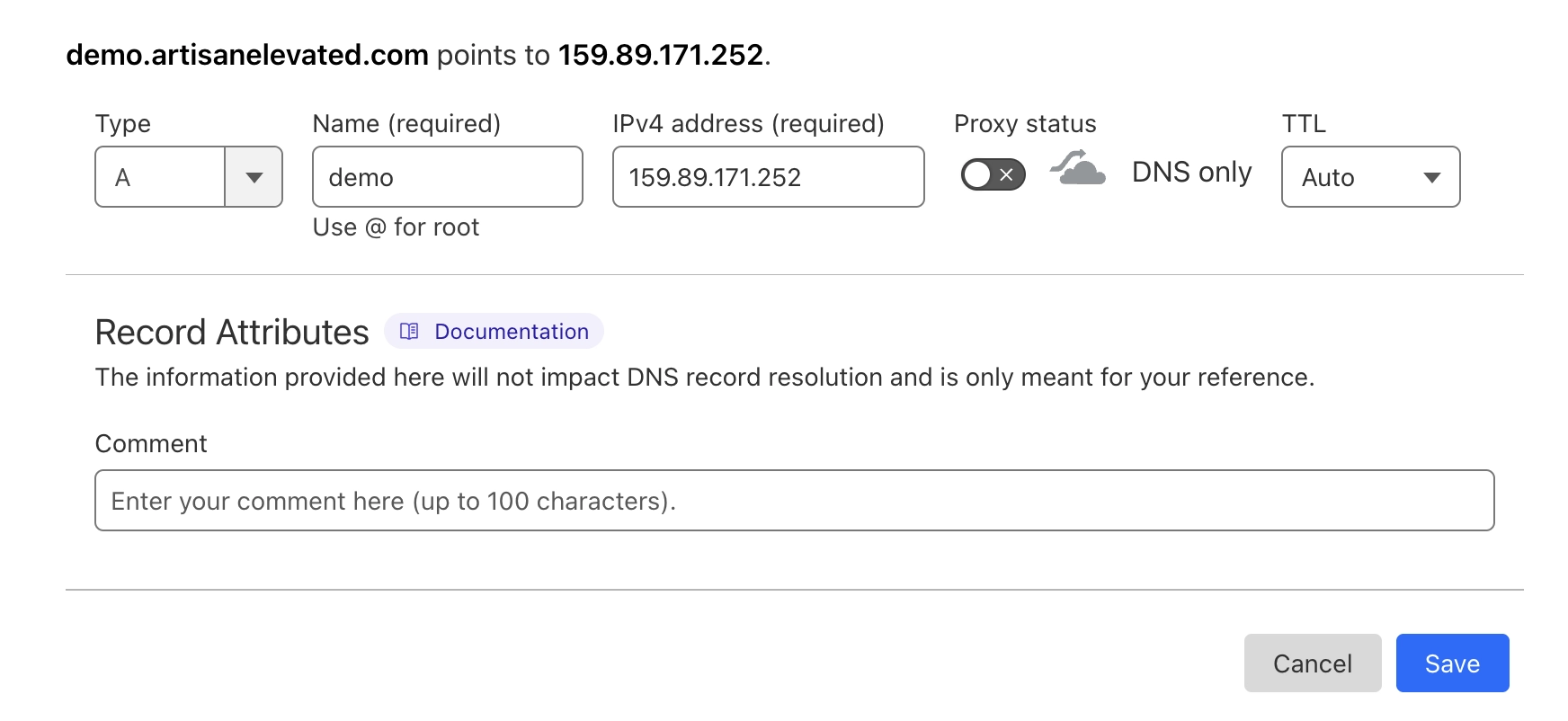
Next we expose the laravel application port to the host so that nginx can proxy requests to it.
shell dokku ports:add laravel-app http:80:8080
nginx.conf Enabling HSTS (using built-in template) Reloading nginx ```
</Tab>
</Tabs>
> We are using port 8080 as the http port as specified in the server-sideup-php-docker image. The ports could be different depending on the image you are using.
We then add the domain to the app. I this process, dokku will automate nginx to proxy requests to the app container. So, no need for your configure the proxy yourself.
<Tabs>
<Tab
name="add-domain"
label="Add Domain"
checked
>
shell dokku domains:add laravel-app demo.artisanelevated.com dokku domains:set laravel-app demo.artisanelevated.com
</Tab>
<Tab
name="add-domain"
label="Output"
>
```shell
-----> Added demo.artisanelevated.com to laravel-app ! Please run dokku letsencrypt:enable to add https
support to the new domain -----> Configuring demo.artisanelevated.com...(using built-in template) ----->
Configuring laravel-app.ubuntu-s-1vcpu-1gb-35gb-intel-blr1-01...(using built-in template) -----> Creating http
nginx.conf Reloading nginx -----> Set demo.artisanelevated.com for laravel-app ! Please run dokku
letsencrypt:enable to add https support to the new domain -----> Configuring demo.artisanelevated.com...(using
built-in template) -----> Creating http nginx.conf Reloading nginx
At this point, you should be able to access the application at http://your-domain.com
. But the application is not secured. Let's secure it.
Step 8 of 9: Secure the domain with Let's Encrypt
Now that we have a domain working, we need to secure it with Let's Encrypt. Start by installing the lets encrypt plugin for dokku.
sudo dokku plugin:install https://github.com/dokku/dokku-letsencrypt.git
Next we enable the lets encrypt plugin for the domain. We will need an email for that.
dokku letsencrypt:set laravel-app email "your-email"
dokku letsencrypt:enable laravel-app
=====> Setting email to [email protected]
=====> Enabling letsencrypt for laravel-app
-----> Enabling ACME proxy for laravel-app...
-----> Getting letsencrypt certificate for laravel-app via HTTP-01
- Domain 'demo.artisanelevated.com'
2024/09/29 23:07:10 [INFO] [demo.artisanelevated.com] Server responded with a certificate.
-----> Certificate retrieved successfully.
-----> Installing let's encrypt certificates
-----> Unsetting DOKKU_PROXY_PORT
-----> Configuring demo.artisanelevated.com...(using built-in template)
-----> Creating https nginx.conf
Enabling HSTS (using built-in template)
Reloading nginx
-----> Ensuring network configuration is in sync for laravel-app
-----> Configuring demo.artisanelevated.com...(using built-in template)
-----> Creating https nginx.conf
Enabling HSTS (using built-in template)
Reloading nginx
-----> Disabling ACME proxy for laravel-app...
-----> Done
Finally we activate a cron job to renew the certificate before it expires.
dokku letsencrypt:cron-job --add
At this point, you should be able to access the application with a secure domain https://your-domain.com
.
If you are using Cloudflare, you can no turn on the orange proxy option and enable strict ssl option.
Step 9 of 9: Start horizon, reverb and scheduler (Optional)
If you application requires horizon or reverb or scheduler, you can start them. To do so, you need to SSH into the server and start the services.
Make sure you have updated the
Procfile
with the correct commands. You only need to start the services once and dokku will take care of the rest in the subsequent deployments.
shell # Create one (1) scheduler container dokku ps:scale laravel-app scheduler=1
shell # Create one (1) horizon container dokku ps:scale laravel-app horizon=1
shell # Create one (1) reverb container dokku ps:scale laravel-app reverb=1
You can also scale the number of workers for the queues. For example, to scale the
web
process to2
workers, rundokku ps:scale laravel-app web=2
. You now have two workers running for the web process. That how easy it is to scale your dokku applications.
With that done, you have your processes running. When you push code to the server, all the containers will be deployed and started.
You can verify that the containers are running by running the following command.
dokku logs laravel-app
If you deploy reverb, you will need to expose port
8080
and add a new websocket domain to the app.
Conclusion
I hope you have learned how easy it is to deploy your Laravel application to the Internet. Using this Dokku to deploy Laravel applications is inexpensive, developer-friendly, and maintainable. Using this knowledge, you can create a staging application for testing. To avoid making this article too long, I did not cover other topics that you should consider researching. These include:
- Backing up Postgres and Redis on S3 compatible object storage.
- Integrating a Dokku CI - GitHub Actions and GitLab CI.
- Mounting storage to the app e.g the laravel storage directory.
- How to manage server ports with
ufw
. - How to debug errors using logs.
If there is any part of this article that is not clear to you, feel free to leave a comment. I will improve it.
Have a look at the source code of this article.
Do you need help deploying your Laravel application? Contact me and we can work it out.